Your ready-to-deploy payouts engine
Use Trolley’s powerful payouts API and out-of-the-box developer tools to automate payout operations, build customized workflows, and grow your platform or marketplace across borders without the heavy lift.
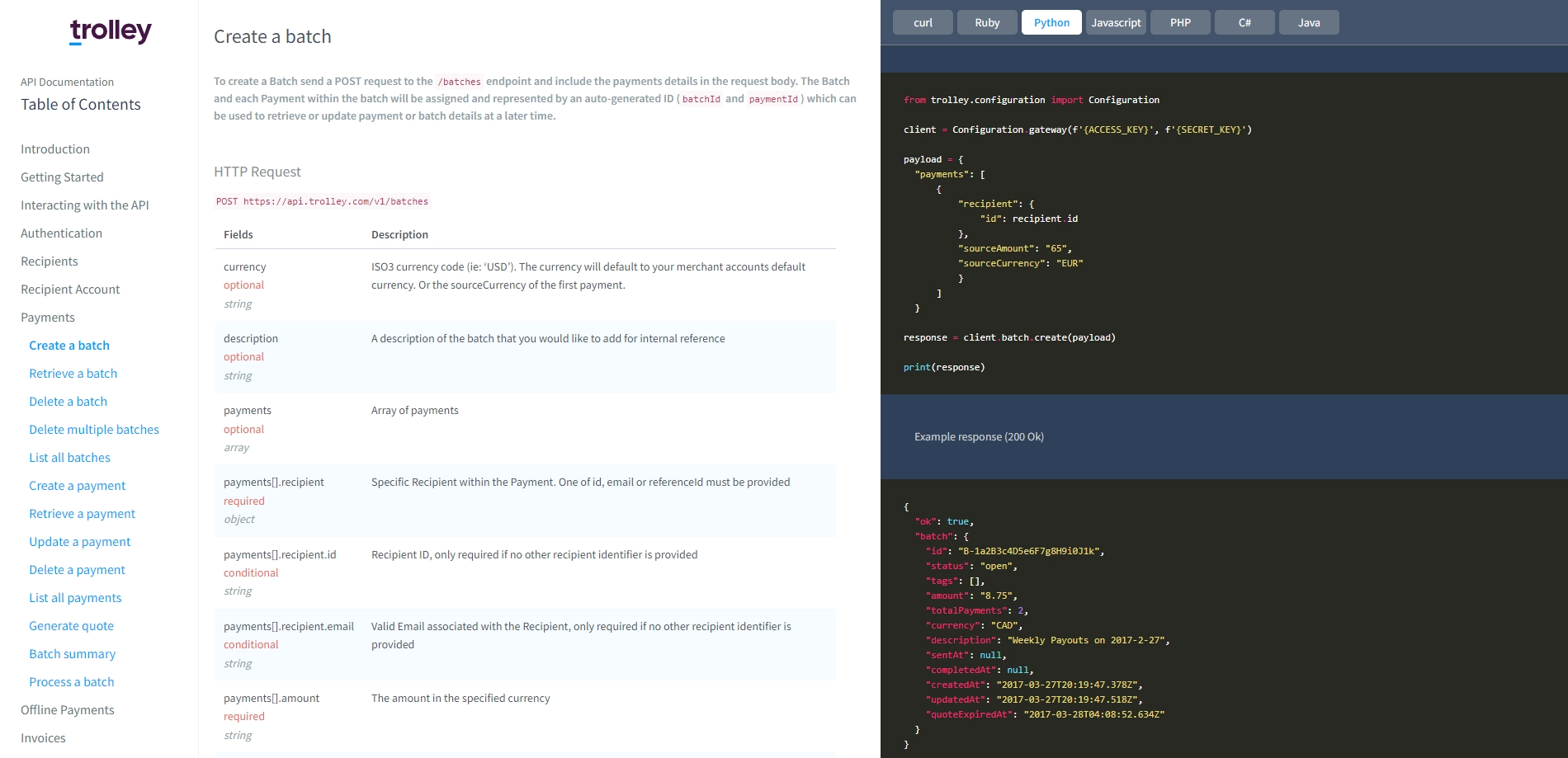
Developer toolkits trusted by enterprises and startups
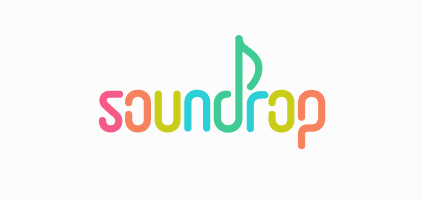
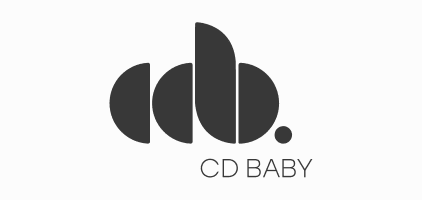
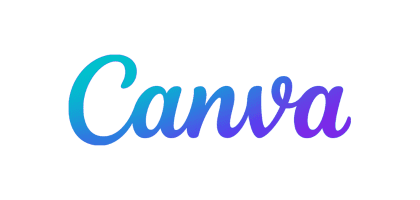
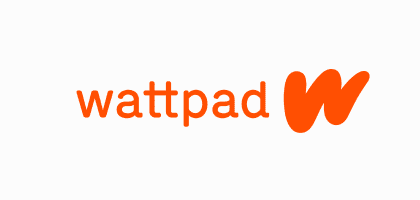
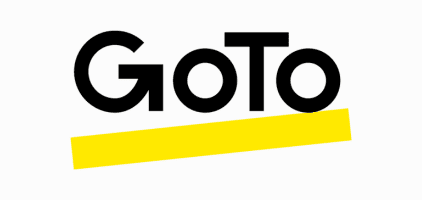
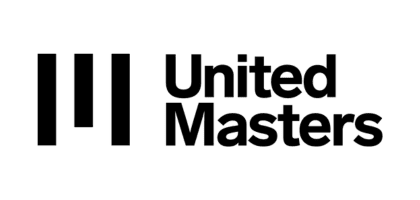
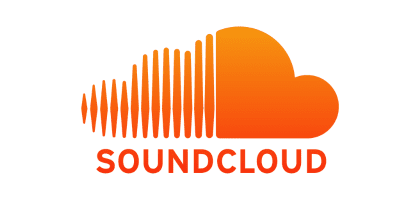
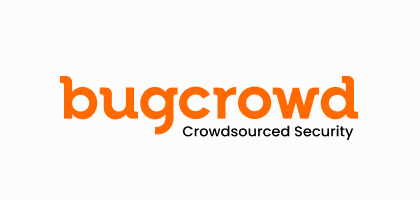
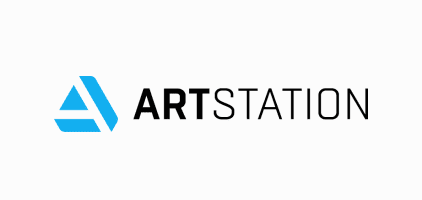
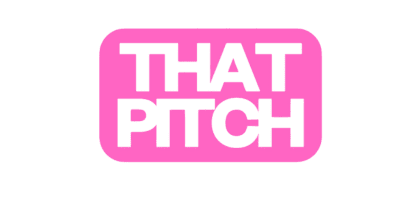
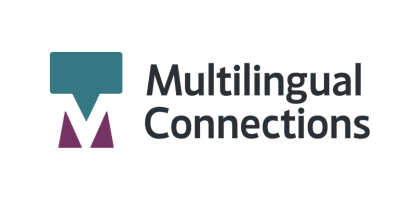
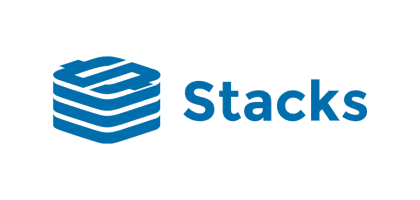
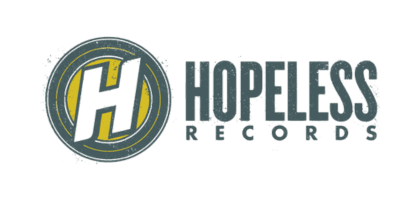
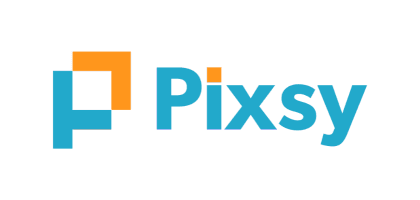
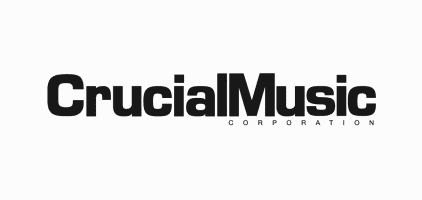
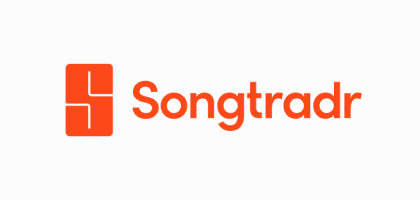
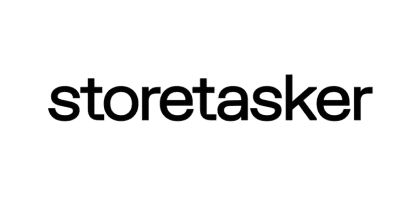
Customized workflows: from sandbox to production in minutes
Built by developers, for developers, Trolley gets that merging payouts infrastructure within your existing code can be challenging. We built our developer tools with this simple premise: devs should be able to move from experimenting in the sandbox to sending payouts in minutes.
2. Choose the tools best suited to your tech stack
3. Play in the free sandbox to build and customize workflows, and test your integration
4. Swap the API keys from sandbox to production
5. You're live!
The global payment platform
It's never been easier to grow your business internationally. Get access to Trolley’s next generation payments infrastructure and global banking network to send funds to suppliers and contractors around the world.
const paymentrails = require('paymentrails');
const client = paymentrails.connect({
key: "YOUR-API-KEY",
secret: "YOUR-API-SECRET",
environment: "production",
});
// Async/Await version
async function main() {
const recipient = await client.recipient.find("R-G7SXXpm6cs4aTUd9YhmgWC");
console.log(recipient.id);
}
main();
// Promise version
client.recipient.find("R-G7SXXpm6cs4aTUd9YhmgWC").then(recipient => {
console.log(recipient.id);
}).catch(err => {
console.log("ERROR", err);
});
from paymentrails.configuration import Configuration
from paymentrails.recipients import Recipients
client = Configuration.gateway("YOUR-PUBLIC-API","YOUR-PRIVATE-API","production")
response = client.recipient.find("R-WJniNq7PUXyboAJetimmJ4")
print(response.id)
require 'payment_rails'
payments = [
{recipient: 1, amount: 100, currency: "USD"},
{recipient: 2, amount: 200, currency: "CAD"}
]
client = PaymentRails.connect(api_key: "")
client.batches.create(recipient)
// This line is for the Composer autoloader
require_once 'vendor/autoload.php';
// Or use this if installed via git clone
// require_once 'php-sdk/lib/autoload.php';
use PaymentRails;
// Configure API key authorization: merchantKey
PaymentRails\Configuration::environment('production');
PaymentRails\Configuration::publicKey(YOUR_PUBLIC_KEY);
PaymentRails\Configuration::privateKey(YOUR_PRIVATE_KEY);
try {
$recipients = PaymentRails\Recipient::all();
foreach ($recipients as $rcpt) {
print_r($rcpt->id . "\n");
}
} catch (Exception $e) {
echo 'Exception when calling PaymentRails\\Recipient::all ', $e->getMessage(), PHP_EOL;
}
using paymentrails.Types;
using paymentrails;
class Program
{
static void Main(string[] args)
{
// set your API key
var client = new PaymentRails_Gateway("YOUR-API-KEY", "YOUR-SECRET-KEY", "production");
Recipient response = client.recipient.find("R-4q7zxMa26hpZhx7ULApBGw");
Console.WriteLine(response.id);
Console.Read();
}
}
Fully flexible APIs
We built Trolley with an API-first philosophy, meaning our platform is powered by our own APIs. Every functionality in the Trolley interface comes with a corresponding API component, enabling you to build workflows tailored to your specific business needs.
Our REST-based APIs:
- Get you up and running in <10 minutes
- Offer extensive scalability
- Cover a wide range of components, allowing you to build sophisticated automations
- Include detailed documentation that guides you through every step of the integration process
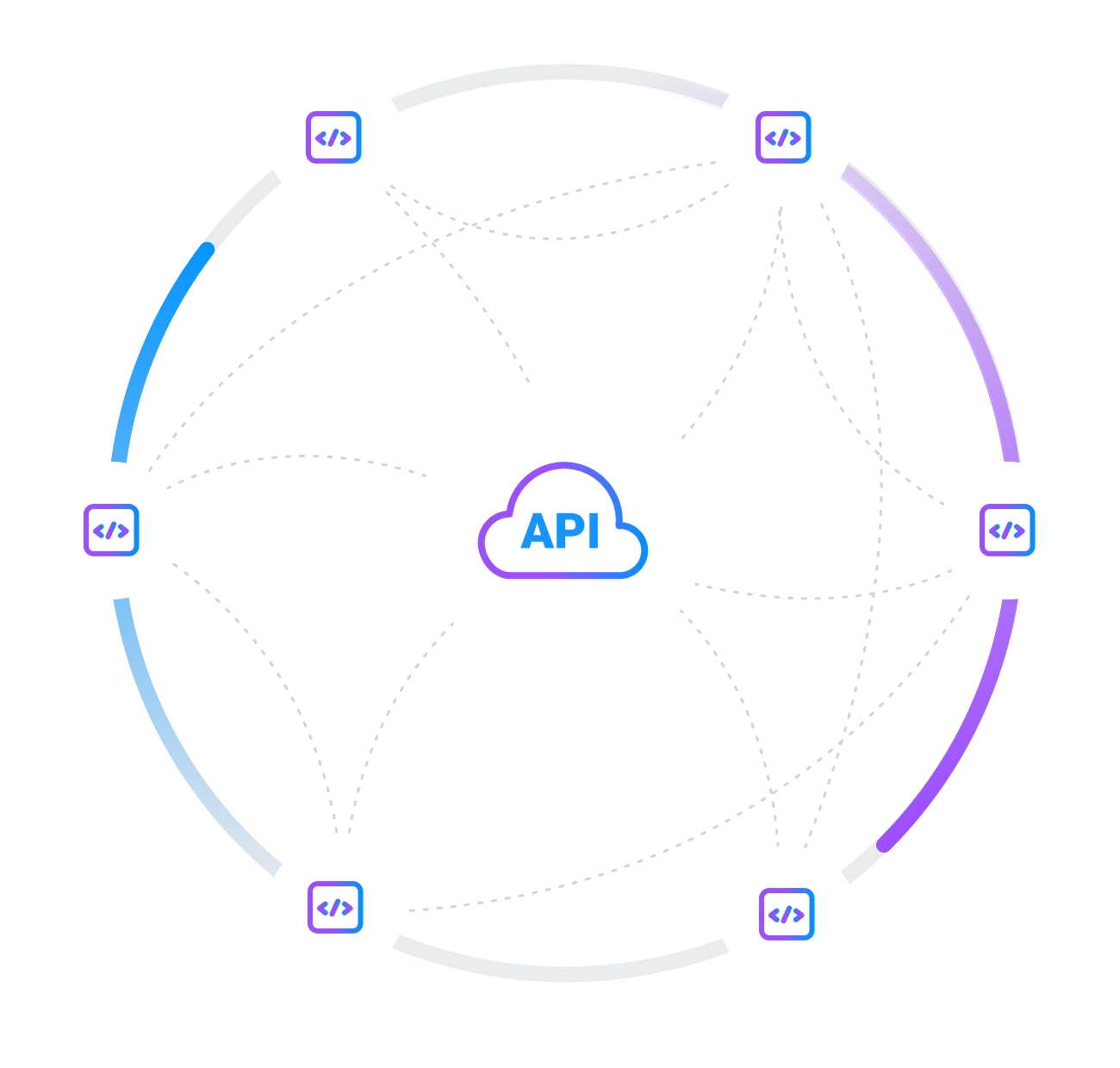
Add fully compliant payouts with pre-packaged tools
Simplify your architecture and skip the hassle of writing hundreds of lines of code with our pre-built tools and libraries.
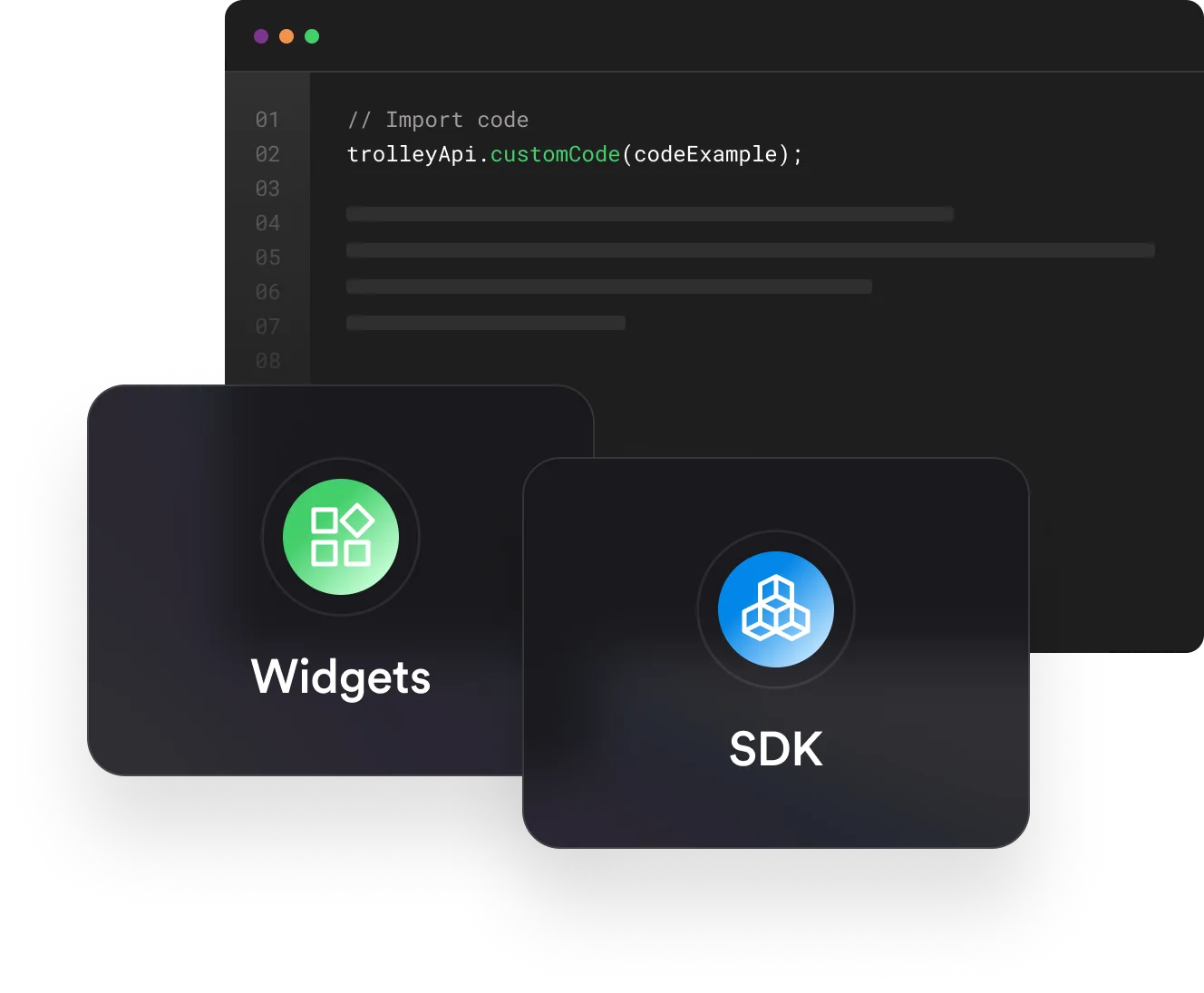
Open source SDKs in popular languages
- JavaScript, Ruby, PHP, Python, Java, and C#
- Available on leading package managers and updated frequently
- Build API functionality natively into your platform
- Benefit from and contribute to open-source projects
Pre-built, modular widget
- White-labeling options to seamlessly integrate with your brand
- Responsive design integrated as an iframe, built-in event emitter
- Plug-and-play functionality for a turnkey solution
- Fully customizable, brandable, and modular
- Choose from different modules—Pay, Tax, and Trust—based on your business needs
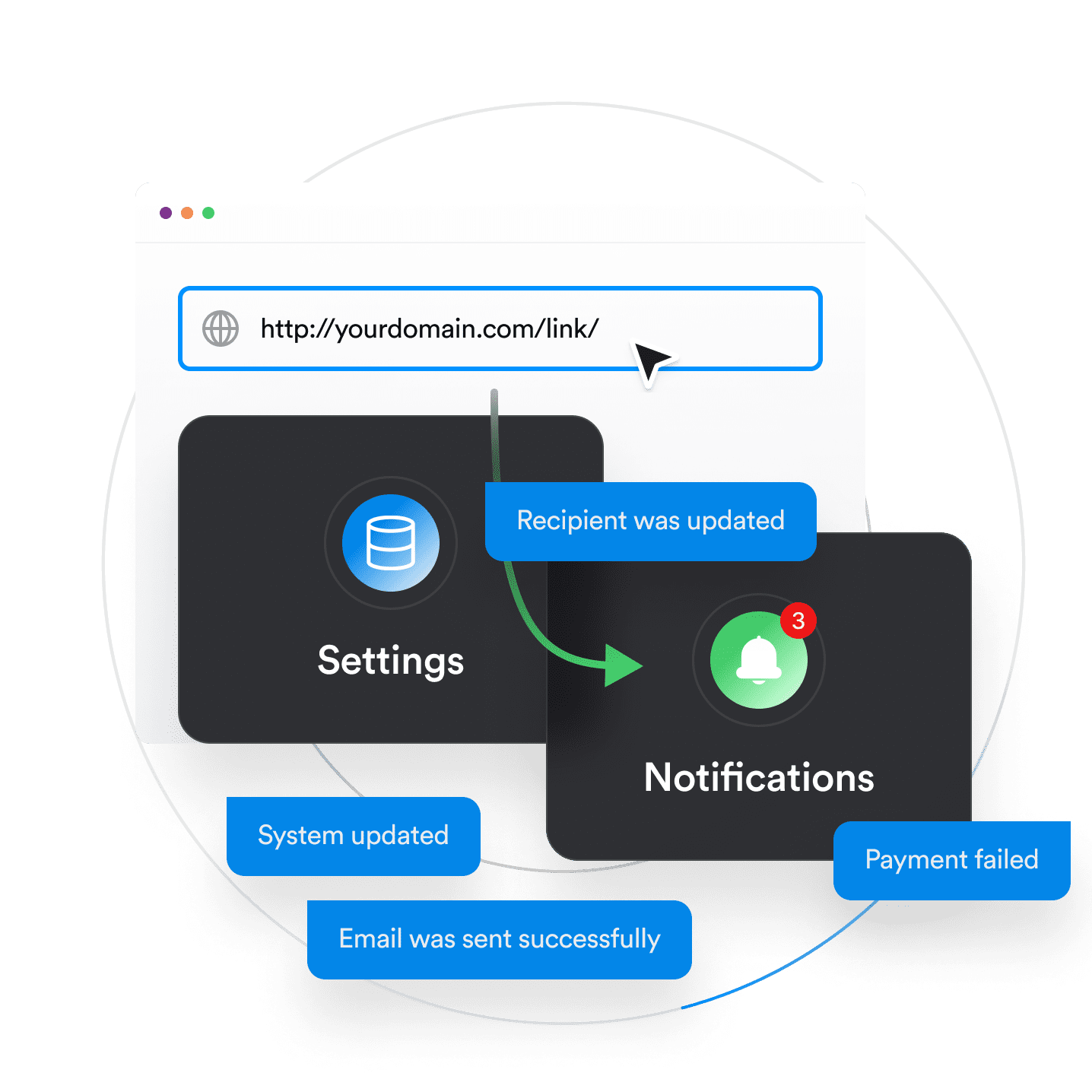
Custom callback webhooks
State-of-the-art, lightweight webhooks that enable communication between your internal apps. Trigger communication with your recipients or update systems per your business logic.
- Receive webhook notifications from us whenever models change (e.g. a recipient was updated, a payment failed, etc.)
- Sequential delivery of webhooks help you respond to events in order
- Reliable and retry-based delivery you can trust
Faster time to market
Fewer lines of code
Bug-free
Launch and iterate faster
Focus on your core workflows and let Trolley handle payouts and compliance. Trolley is built for the internet economy, so you don’t have to choose between scale, speed, and quality.
Postman collection
Test the integration of Trolley’s APIs before you put in the work. Trolley’s Postman collection lets you take us for a test drive without any commitments.
- Full-featured collection covering all our API endpoints
- Automatic handling of Trolley authentication for each request
- Get comfortable with how our APIs behave before coding your solution
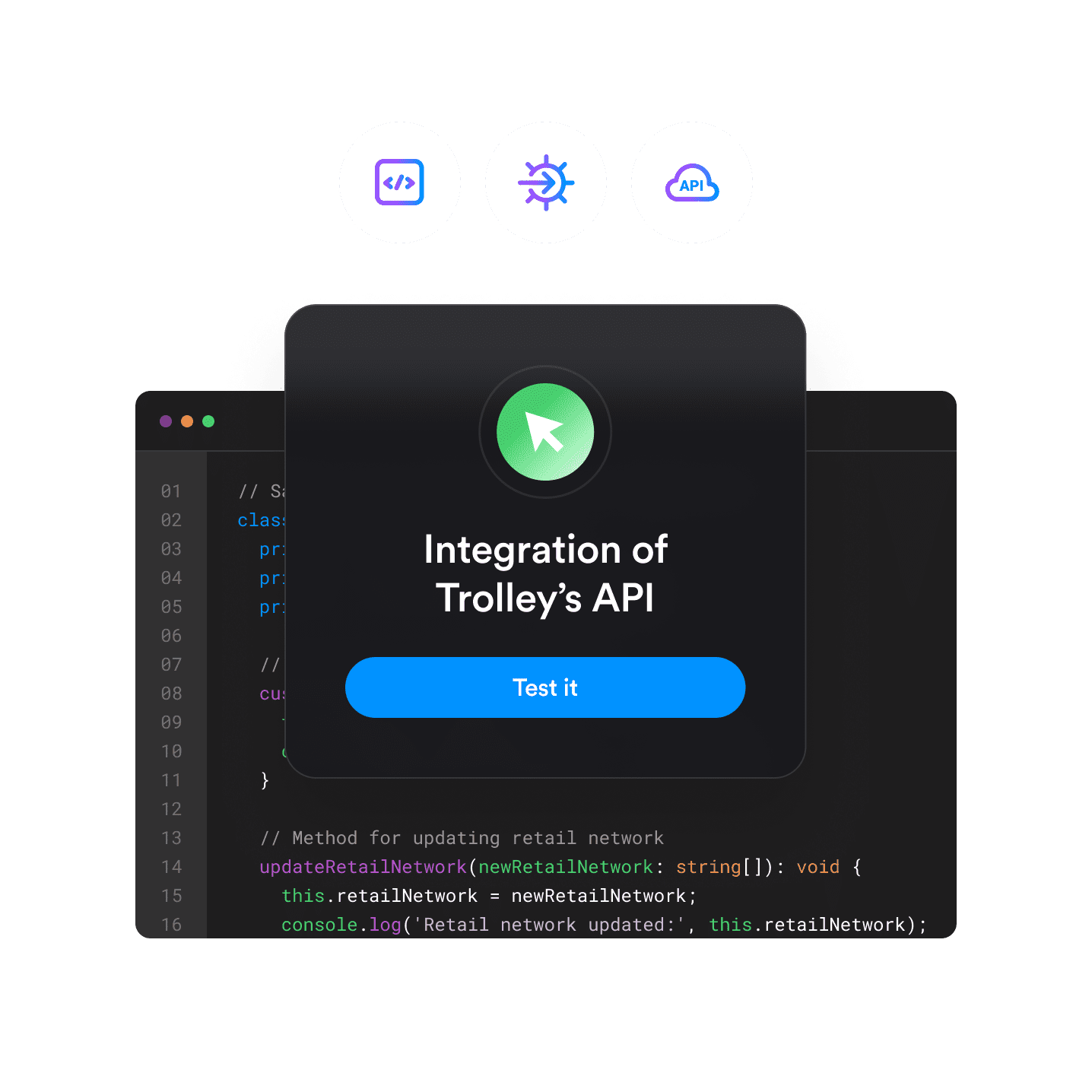
A complete ecosystem for developers
Providing a fully integrated user experience for your recipients doesn’t need to be complicated. Use our full range of developer tools to build the integrations you want, with accessible support teams always ready to help.
A spectrum of tools, from one-line integrations to full customization
An always-free, self-serve sandbox lets you build, customize, and test before you decide to buy
Comprehensive documentation, including developer guides and blogs to help you get the most out of our tools
Open-source contributions and an advocacy team that is constantly improving based on developer feedback
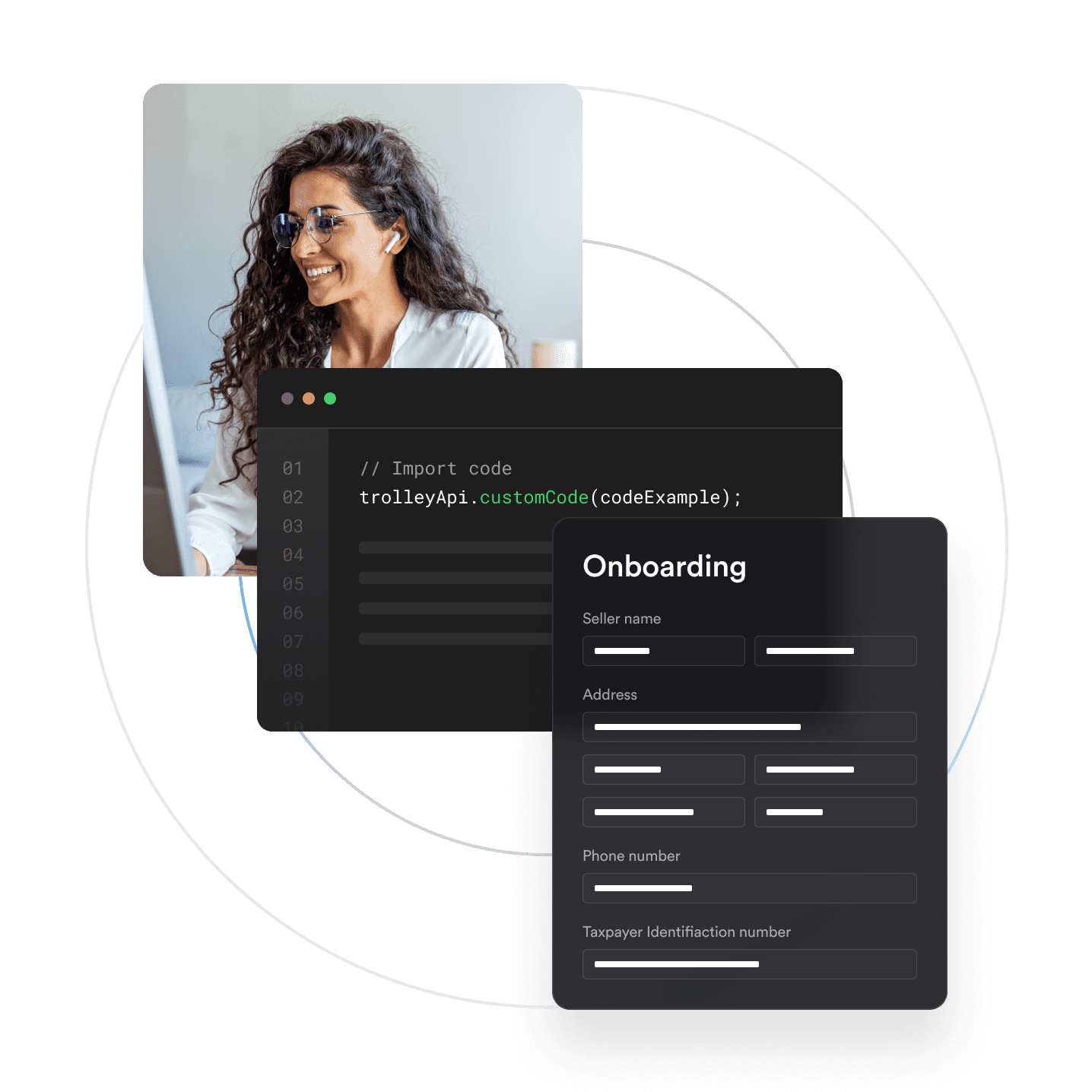
Best-in-class developer and recipient experience
Some platforms are dev-friendly, while others focus on the end-user experience. Trolley understands that both are a competitive advantage in the internet economy.
- Best-in-class recipient experience with modern UX, reducing drop-offs
- Detailed documentation covering all aspects of our API offering
- Full-featured sandbox environment where you can test as much as you want
- Support teams ready to assist you at every stage of the integration process
- Easy import of your recipient data to help you get started